Reactive Box
Today I had great success with implementing some basic reactive functionality.
At the basis of the editor UI there is a really crude reactive library I wrote. The idea is that you create boxes for values, and you call a getter to retrieve the value, but every time you call it, a dependency is recorded. There’s also a compute function which takes a computation function as argument, and takes the dependencies recorded during the execution of the computation and sets things up so when any dependency box’s content changes the whole computation function is re-run. This computation function should have some sort of side-effect, e.g. setting another box’s value or updating a DOM element.
This method results in a lot of noise in the code for setting up each box. Now, as I’m in the process of reimplementing the UI with itself, it occurred to me that I can just add a new language element for defining a box. I can set the type of a class property to a box and then just use it as a normal variable, and let the transpiler insert all the repetitive code that makes it work.
This now works for my little contrived example: I have a class with three reactive box properties; x
is initialised through the constructor and can also be set from the outside. Whenever x
changes, y
will be automatically set to x*5
. When y
changes, z
is set to the string "Z:"+y
. Whenever z
changes, the compute
block on the top updates the DOM. As I set the value of x
to 0
, 1
, 2
, the DOM shows Z:0
, Z:5
, Z:10
, etc.
Whilelse code rendering:
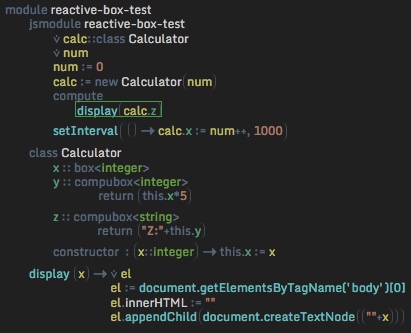
1 | function box() { |